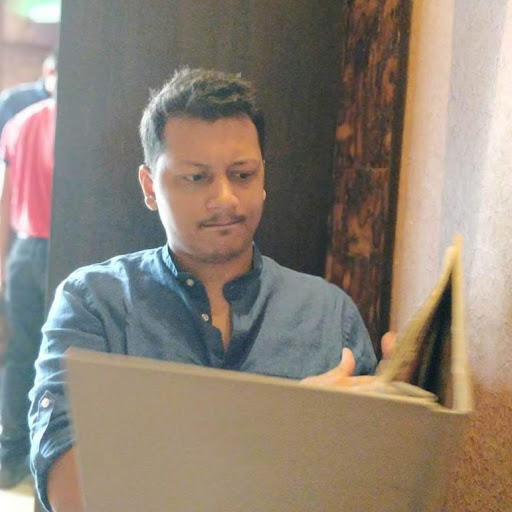
Cron Jobs with Django and GitHub Actions
Railway now supports Cron Jobs natively. It may be a better fit for you than what’s written here. Check out https://docs.railway.app/reference/cron-jobs for details!
We’ve been asked a number of times how to orchestrate a cron job on Railway. Today we’re going to show you how to create your first Django cron using GitHub Actions.
This may come in handy if you’re looking to add a cron to a new or existing Railway project and will make it possible to run any Django task on a schedule on Railway.
Let’s get into it!
For those of you who are here to grab the example code, here’s the entire YAML needed to create a GitHub Action to run your Django tasks on a cron:
name: My Command
on:
schedule:
- cron: '*/4 * * * *'
jobs:
build:
name: Run crons
runs-on: ubuntu-latest
steps:
- uses: actions/checkout@v3
- uses: actions/setup-node@v3
with:
node-version: 16
- name: Install Railway
run: npm i -g @railway/cli
- name: Install Python packages
run: railway run --service {{SERVICE_ID}} pip install -r requirements.txt
env:
RAILWAY_TOKEN: ${{ secrets.RAILWAY_TOKEN }}
- name: My Command
run: railway run --service {{SERVICE_ID}} python manage.py my_command
env:
RAILWAY_TOKEN: ${{ secrets.RAILWAY_TOKEN }}
If you care to understand a bit more about how this works, please read on.
In the snippet below, we’re telling GitHub to run this Action every 4 minutes.
on:
schedule:
- cron: '*/4 * * * *'
Of course, you may use any cron expression you like to run your Action at your preferred interval. We recommend checking out CrontabGuru, which is a handy service to test out your cron expressions before adding them to your Action.
Next, we’ll install the Railway CLI which will allow us to interact with our Railway project from within the GitHub Action. You can read the docs to learn more about the CLI.
name: Install Railway
run: npm i -g @railway/cli
Now that the Railway CLI is installed, we’ll run the task.
In the snippet below, we’ll invoke our my_command
management command on our service using the Railway CLI. If you don’t have a RAILWAY_TOKEN
secret, note that this can be generated by creating a Project Token for your project.
name: My Command
run: railway run --service {{SERVICE_ID}} python manage.py my_command
env:
RAILWAY_TOKEN: ${{ secrets.RAILWAY_TOKEN }}
Success! We’ve now run the task and started the cron!
In a few simple steps, we’ve made it possible to execute any Django task at a specific interval on our Railway projects and services.
We’d like to give a shoutout to Dan Rowden for sharing this GitHub Action which was written for a new project called InstantDM.
Additionally, if someone in the community would like re-work this GitHub Action to run rake tasks for Ruby on Rails — reach out and let us know! We’d love to help you get it working.